TLDR: Generate FAQ schema markups for your website with this Python script that takes input from a spreadsheet and produces a JSON file following the FAQPage schema of schema.org in just a few seconds.
As an SEO professional, you understand the importance of having a website that is easily discoverable by search engines. One way to achieve this is by using structured data in the form of schema.org.
Recently, I created a tool that makes it easy to generate FAQ schema markups for websites. This tool takes input from a spreadsheet with two columns, one for questions and one for answers, and then generates a JSON file that follows the FAQPage schema of schema.org.
By providing a clear and organized format for your FAQs, search engines can understand your website’s content better and show it in search results more effectively. This can improve your website’s SEO and provide more detailed and relevant results for users.
The script uses the popular libraries pandas to read the spreadsheet and extract the questions and answers from it, and json to generate the json file.
First, the script starts by importing the required libraries:
import pandas as p
import json
Then, it reads the spreadsheet with questions and answers. The script assumes that the spreadsheet has two columns, one for questions and one for answers, and that the first row contains the column headers.
df = pd.read_excel("name-of-the-sheet.xlsx")
After reading the spreadsheet, the script creates a dictionary for the FAQ schema and sets its context and type.
faq_schema = {
"@context": "http://schema.org",
"@type": "FAQPage",
"mainEntity": []
}
Then, it iterates over each row in the spreadsheet, gets the question and answer from the row, creates a dictionary for the question-answer pair.
for index, row in df.iterrows():
question = row["question"]
answer = row["answer"]
question_answer = {
"@type": "Question",
"name": question,
"acceptedAnswer": {
"@type": "Answer",
"text": answer
}
}
and then appends it to the mainEntity of the faq_schema.
faq_schema["mainEntity"].append(question_answer)
Finally, the script saves the FAQ schema to a JSON file named faq-schema.json using the json.dump method.
with open('faq-schema.json', 'w') as json_file
json.dump(faq_schema, json_file, indent=4)
An example of input spreadsheet that the script can take as input:
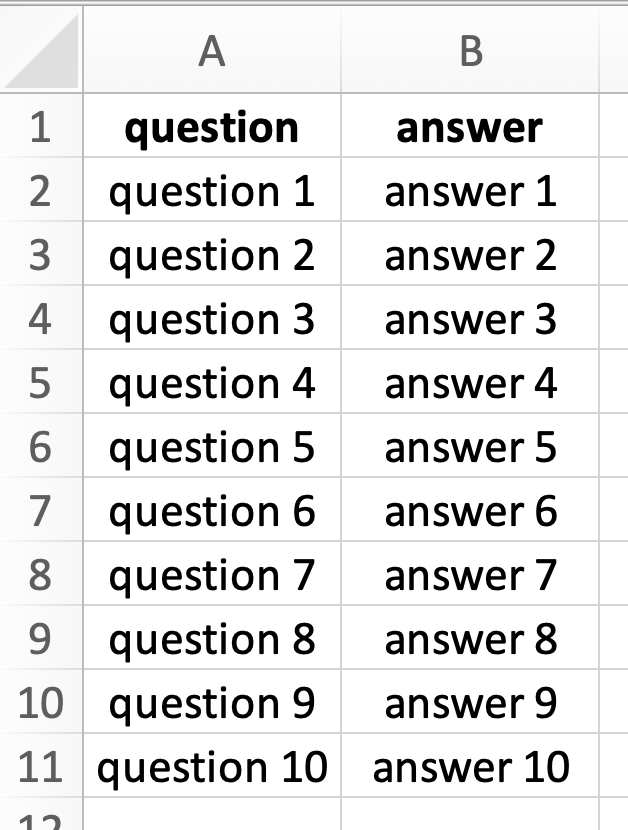
An example of output that the script generates:
{
"@context": "http://schema.org",
"@type": "FAQPage",
"mainEntity": [
{
"@type": "Question",
"name": "question 1",
"acceptedAnswer": {
"@type": "Answer",
"text": "answer 1"
}
},
{
"@type": "Question",
"name": "question 2",
"acceptedAnswer": {
"@type": "Answer",
"text": "answer 2"
}
},
{
"@type": "Question",
"name": "question 3",
"acceptedAnswer": {
"@type": "Answer",
"text": "answer 3"
}
},
{
"@type": "Question",
"name": "question 4",
"acceptedAnswer": {
"@type": "Answer",
"text": "answer 4"
}
},
{
"@type": "Question",
"name": "question 5",
"acceptedAnswer": {
"@type": "Answer",
"text": "answer 5"
}
},
{
"@type": "Question",
"name": "question 6",
"acceptedAnswer": {
"@type": "Answer",
"text": "answer 6"
}
},
{
"@type": "Question",
"name": "question 7",
"acceptedAnswer": {
"@type": "Answer",
"text": "answer 7"
}
},
{
"@type": "Question",
"name": "question 8",
"acceptedAnswer": {
"@type": "Answer",
"text": "answer 8"
}
},
{
"@type": "Question",
"name": "question 9",
"acceptedAnswer": {
"@type": "Answer",
"text": "answer 9"
}
},
{
"@type": "Question",
"name": "question 10",
"acceptedAnswer": {
"@type": "Answer",
"text": "answer 10"
}
}
]
}
In summary, this tool is a simple and efficient way for SEOs to generate FAQ schema markups for their website. It takes input from a spreadsheet and generates a JSON file that follows the FAQPage schema of schema.org.
If you have any questions, feel free to comment here or message me, I’ll be happy to help you. If you want to learn more on how to advance your technical SEO skills with Python, be sure to follow me.